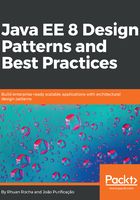
Implementing the business-object pattern
We are now going to input some code in order to illustrate the business-object pattern. However, we must again pay attention to the fact that there is likely another approach to getting the results. For instance, we could use an O-R Mapping (JPA or Hibernate technology) to map the entities.
As an example, the Professor entity has an n-to-n relationship with the Discipline entity, which is done with a JPA annotation. However, we know that there are many more use cases here than simply mapping entities.
We will use ProfessorBO, Professor, Discipline, ProfessorDAO, and DisciplineDAO. Let's take advantage of the classes shown in the Session Fa?ade example. We made a small change in the AcademicFacadeImpl class. Now, this Session Fa?ade uses a BO called ProfessorBO to handle the business related to Professor.
Let's review the ProfessorBO class:
import java.time.LocalDate;
import java.util.List;
import javax.inject.Inject;
public class ProfessorBO {
private Professor professor;
private List<Discipline> disciplines;
@Inject
private ProfessorDAO professorDAO;
@Inject
private DisciplineDAO disciplineDAO;
public void setProfessor (Professor professor ) {
this.professor = professorDAO.findByName (professor.getName());
}
public boolean canTeachDiscipline (Discipline discipline) {
if (disciplines == null) {
disciplines = disciplineDAO.getDisciplinesByProfessor (professor);
}
return disciplines.stream().anyMatch(d->d.equals(discipline));
//return disciplines.contains(discipline);
}
public LocalDate getInitDate () {
return professor.getInitDate();
}
public String getName () {
return professor.getName();
}
}
Let's also have a look at the AcademicFacadeImpl class:
@Stateless
@LocalBean
public class AcademicFacadeImpl implements AcademicFacadeRemote, AcademicFacadeLocal {
...
...
@Inject
private ProfessorBO professorBO;
@Override
public List<Professor> getProfessorsByDiscipline(Discipline discipline) {
return disciplineDAO.getProfessorByDiscipline(discipline);
}
public boolean canProfessorTeachDiscipline (Professor professor, Discipline discipline) {
/*return disciplineDAO.getDisciplinesByProfessor (professor).contains(discipline);*/
professorBO.setProfessor (professor);
return professorBO.canTeachDiscipline(discipline);
}
}
As we can see in the preceding code block, the AcademicFacadeImpl Session Fa?ade calls the canTeachDiscipline method from the ProfessorBO injected bean. ProfessorBO then uses ProfessorDAO and DisciplineDAO. Next, we will see the part of the DisciplineDAO code that is used by the ProfessorBO bean:
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class DisciplineDAO {
static {
Discipline d1 = new Discipline("D1", "discipline 1");
Discipline d2 = new Discipline("D2", "discipline 2");
Discipline d3 = new Discipline("D3", "discipline 3");
Discipline d4 = new Discipline("D4", "discipline 4");
disciplines = Arrays.asList(d1, d2, d3, d4);
...
professorXDisciplines.put (new Professor ("professor a"), Arrays.asList (d1, d2));
professorXDisciplines.put (new Professor ("professor b"), Arrays.asList (d3));
professorXDisciplines.put (new Professor ("professor cv"), Arrays.asList (d1, d3, d4));
}
...
public List<Discipline> getDisciplinesByProfessor(Professor professor) {
return professorXDisciplines.get (professor);
}
...
}
Let's see the code used by the ProfessorDAO class:
public class ProfessorDAO {
private static Set<Professor> professors;
static {
Professor p1 = new Professor ("professor a", LocalDate.of (2001, 03,
22)),
p2 = new Professor ("professor b", LocalDate.of (1994, 07, 05)),
p3 = new Professor ("professor c", LocalDate.of (1985, 10, 12)),
p4 = new Professor ("professor cv", LocalDate.of (2005, 07, 17));
professors = Arrays
.stream (new Professor[]{p1, p2, p3, p4})
.collect (Collectors.toSet());
}
public Professor findByName (String name) {
return professors
.stream()
.filter(p->p.getName().equals(name))
.findAny()
.get();
}
}
Finally, let's see the ProfessorBO class:
import java.time.LocalDate;
import java.util.List;
import javax.inject.Inject;
public class ProfessorBO {
private Professor professor;
private List<Discipline> disciplines;
@Inject
private ProfessorDAO professorDAO;
@Inject
private DisciplineDAO disciplineDAO;
public void setProfessor (Professor professor ) {
this.professor = professorDAO.findByName (professor.getName());
}
public boolean canTeachDiscipline (Discipline discipline) {
if (disciplines == null) {
disciplines = disciplineDAO.getDisciplinesByProfessor
(professor);
}
return disciplines.stream().anyMatch(d->d.equals(discipline));
//return disciplines.contains(discipline);
}
public LocalDate getInitDate () {
return professor.getInitDate();
}
public String getName () {
return professor.getName();
}
}