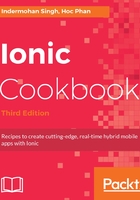
上QQ阅读APP看书,第一时间看更新
How to do it...
Here are the instructions to create example app:
- Create a new LeftRightMenu app using the sidemenu template, as shown, and go to the LeftRightMenu folder:
$ ionic start LeftRightMenu sidemenu $ cd LeftRightMenu
- Check that your app folder structure is similar to the following:

- Edit ./src/app/app.component.ts and replace it with the following code:
import { Component, ViewChild } from '@angular/core';
import { Nav, Platform } from 'ionic-angular';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { HomePage } from '../pages/home/home';
import { ListPage } from '../pages/list/list';
@Component({
templateUrl: 'app.html'
})
export class MyApp {
@ViewChild(Nav) nav: Nav;
text: string = '';
rootPage: any = HomePage;
pages: Array<{title: string, component: any}>;
constructor(public platform: Platform, public statusBar: StatusBar, public splashScreen: SplashScreen) {
this.initializeApp();
// used for an example of ngFor and navigation
this.pages = [
{ title: 'Home', component: HomePage },
{ title: 'List', component: ListPage }
];
}
initializeApp() {
this.platform.ready().then(() => {
// Okay, so the platform is ready and our plugins are available.
// Here you can do any higher level native things you might need.
this.statusBar.styleDefault();
this.splashScreen.hide();
});
}
openPage(page) {
// Reset the content nav to have just this page
// we wouldn't want the back button to show in this scenario
this.nav.setRoot(page.component);
}
rightMenuClick(text) {
this.text = text;
}
}
- Open and edit the./src/app/app.html file with the following code:
<ion-menu id="leftMenu" [content]="content" side="left" type="overlay">
<ion-header>
<ion-toolbar>
<ion-title>Menu</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-list>
<button menuClose ion-item *ngFor="let p of pages"
(click)="openPage(p)">
{{p.title}}
</button>
</ion-list>
</ion-content>
</ion-menu>
<ion-menu id="rightMenu" [content]="content" side="right" type="reveal">
<ion-header>
<ion-toolbar>
<ion-title>Items</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-list>
<button ion-item (click)="rightMenuClick('Item One')">
Item One
</button>
<button ion-item (click)="rightMenuClick('Item Two')">
Item Two
</button>
</ion-list>
<ion-card *ngIf="text">
<ion-card-content>
You just clicked {{ text }}
</ion-card-content>
</ion-card>
</ion-content>
</ion-menu>
<!-- Disable swipe-to-go-back because it's poor UX to combine STGB with side menus -->
<ion-nav [root]="rootPage" #content swipeBackEnabled="false"></ion-nav>
There are two menus as siblings in this template. They are also at the same level as ion-nav and not a parent or child. This structure is important for menu navigation to work.
- Now let's create two pages, for which you only have to modify the standard pages from the sidemenu template. Open and edit the ./src/app/pages/home/home.html template:
<ion-header>
<ion-navbar>
<ion-title>Getting Started</ion-title>
<ion-buttons left>
<button ion-button menuToggle="leftMenu">
<ion-icon name="menu"></ion-icon>
</button>
</ion-buttons>
<ion-buttons right>
<button ion-button menuToggle="rightMenu">
<ion-icon name="menu"></ion-icon>
</button>
</ion-buttons>
</ion-navbar>
</ion-header>
<ion-content padding class="getting-started">
<h3>Welcome to the Menu Experiment</h3>
<p>
You can open both left and right menu using below buttons or top
navigation bar!
</p>
<ion-row>
<ion-col width-50>
<button ion-button primary block menuToggle="leftMenu">Toggle Left</button>
</ion-col>
<ion-col width-50>
<button ion-button primary block menuToggle="rightMenu">Toggle Right</button>
</ion-col>
</ion-row>
</ion-content>
- In the same folder, open and edit the .css classes via home.scss, as shown:
page-home {
.getting-started {
p {
margin: 20px 0;
line-height: 22px;
font-size: 16px;
}
}
.bar-button-menutoggle {
display: inline-flex;
}
}
Note that since you're using the sidemenu template, it already comes with a second page (for example, list). There is no need to modify that page in this specific example.
- Open and edit the template for the second page at ./src/pages/list/list.html, as shown:
<ion-header>
<ion-navbar>
<button ion-button menuToggle>
<ion-icon name="menu"></ion-icon>
</button>
<ion-title>List</ion-title>
</ion-navbar>
</ion-header>
<ion-content>
<ion-list>
<button ion-item *ngFor="let item of items" (click)="itemTapped($event, item)">
<ion-icon [name]="item.icon" item-left></ion-icon>
{{item.title}}
<div class="item-note" item-right>
{{item.note}}
</div>
</button>
</ion-list>
<div *ngIf="selectedItem" padding>
You navigated here from <b>{{selectedItem.title}}</b>
</div>
</ion-content>
- Go to your Terminal and run the app:
$ ionic serve