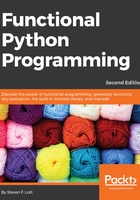
Using a functional hybrid
We'll continue this example with a mostly functional version of the previous example to compute the sum of multiples of three and five. Our hybrid functional version might look like the following:
print(sum(n for n in range(1, 10) if n%3==0 or n%5==0))
We've used nested generator expressions to iterate through a collection of values and compute the sum of these values. The range(1, 10) method is iterable and, consequently, a kind of generator expression; it generates a sequence of values . The more complex expression n for n in range(1, 10) if n%3==0 or n%5==0 is also an iterable expression. It produces a set of values,
. The variable n is bound to each value, more as a way of expressing the contents of the set than as an indicator of the state of the computation. The sum() function consumes the iterable expression, creating a final object, 23.
The if clause of the expression can be extracted into a separate function, allowing us to easily repurpose this for other rules. We could also use a higher-order function named filter() instead of the if clause of the generator expression. We'll save this for Chapter 5, Higher-Order Functions.
The variable n in this example isn't directly comparable to the variable n in the first two imperative examples. A for statement (outside a generator expression) creates a proper variable in the local namespace. The generator expression does not create a variable in the same way as a for statement does:
>>> sum(n for n in range(1, 10) if n%3==0 or n%5==0) 23 >>> n Traceback (most recent call last): File "<stdin>", line 1, in <module> NameError: name 'n' is not defined
The variable n doesn't exist outside the binding in the generator expression. It doesn't define the state of the computation.