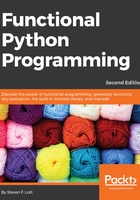
Combining generator expressions
The essence of functional programming comes from the ways we can easily combine generator expressions and generator functions to create very sophisticated composite processing sequences. When working with generator expressions, we can combine generators in several ways.
One common way to combine generator functions is when we create a composite function. We may have a generator that computes (f(x) for x in range()). If we want to compute g(f(x)), we have several ways to combine two generators.
We can tweak the original generator expression as follows:
g_f_x = (g(f(x)) for x in range())
While technically correct, this defeats any idea of reuse. Rather than reusing an expression, we rewrote it.
We can also substitute one expression within another expression, as follows:
g_f_x = (g(y) for y in (f(x) for x in range()))
This has the advantage of allowing us to use simple substitution. We can revise this slightly to emphasize reuse, using the following commands:
f_x = (f(x) for x in range()) g_f_x = (g(y) for y in f_x)
This has the advantage of leaving the initial expression, (f(x) for x in range()), essentially untouched. All we did was assign the expression to a variable.
The resulting composite function is also a generator expression, which is also lazy. This means that extracting the next value from g_f_x will extract one value from f_x, which will extract one value from the source range() function.