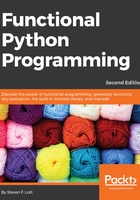
Using the iter() function explicitly
The purely functional viewpoint is that all of our iterables can be processed with recursive functions, where the state is merely the recursive call stack. Pragmatically, Python iterables will often involve evaluation of other for loops. There are two common situations: collection objects and iterables. When working with a collection object, an iterator object is created by the for statement. When working with a generator function, the generator function is an iterator and maintains its own internal state. Often, these are equivalent, from a Python programming perspective. In rare cases—generally those situations where we have to use an explicit next() function—two won't be precisely equivalent.
The legs() function shown previously has an explicit next() evaluation to get the first value from the iterable. This works wonderfully well with generator functions, expressions, and other iterables. It doesn't work with sequence objects such as tuples or lists.
The following code contains three examples to clarify the use of the next() and iter() functions:
>>> list(legs(x for x in range(3))) [(0, 1), (1, 2)] >>> list(legs([0,1,2])) Traceback (most recent call last): File "<stdin>", line 1, in <module> File "<stdin>", line 2, in legs TypeError: 'list' object is not an iterator >>> list(legs(iter([0,1,2]))) [(0, 1), (1, 2)]
In the first case, we applied the legs() function to an iterable. In this case, the iterable was a generator expression. This is the expected behavior based on our previous examples in this chapter. The items are properly paired up to create two legs from three waypoints.
In the second case, we tried to apply the legs() function to a sequence. This resulted in an error. While a list object and an iterable are equivalent when used in a for statement, they aren't equivalent everywhere. A sequence isn't an iterator; it doesn't implement the next() function. The for statement handles this gracefully, however, by creating an iterator from a sequence automatically.
To make the second case work, we need to explicitly create an iterator from a list object. This permits the legs() function to get the first item from the iterator over the list items. The iter() function will create an iterator from a list.