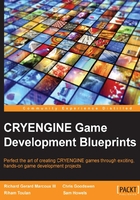
上QQ阅读APP看书,第一时间看更新
Important classes within the starter-kit
As we have concluded the setting up of the starter-kit, it is probably a good time to bring up a few disclaimers:
- While starter-kits in general are extremely helpful, they are technically not a true blank slate.
- There are a few interfaces and classes that will need to be explained before writing code.
- Since there is absolutely no gameplay-specific or genre-specific code or classes, the gameplay mechanics and many other things must be implemented from scratch. Although I consider this to be a good thing, some may not.
To address the previous concerns, let's take a closer look at some of the more important classes within the starter-kit that we will be using on a regular basis, keeping in mind that the names of these classes will be different for you as they are based on the project's name:
CEditorGASGame
: This class implements CRYENGINE'sIEditorGame
interface. It is used to create, update, and shut down your game while you are running it inside the CRYENGINE Sandbox Editor. You often need your game to behave differently while testing inside the editor; this class allows us to do just that. It acts as a proxy for our game, giving us the ability to add or remove functionality that should only exist while playing your game in the editor. For now, we simply forward most calls to the actualGame
class so that our game behaves similarly in the editor and in the Launcher.CGASGame
: This class implements CRYENGINE'sIGame
interface. It is used to update your game and the rest of CRYENGINE's systems. It acts as a hub that manages every system that your game will use, controls communication between them, and facilitates all of the core mechanics of your game. You can view this class as your game's manager—something that orchestrates all of the little moving parts of your game. For now, we simply update the Game Framework, which, in turn, updates the rest of CRYENGINE's systems such as the Renderer, Input Manager, and Physics System.CGASGameRules
: This class implements CRYENGINE'sIGameRules
interface. It is used to carry out your game's rules and works hand-in-hand with yourGame
class. It is a class that does and should receive notifications about any gameplay-specific events so that it may decide how best to handle them in accordance with your game's rules. It is also common for this class to dispatch gameplay-specific events to yourGame
class. For now, this class simply creates the player when asked to do so.CGASModule
: This class is a completely custom class in that it doesn't derive from any CRYENGINE interface. I created this class to help you; by definition, it is a helper class. Its sole purpose is to provide project-wide access to all of your game's systems and instantiate them only when needed. It does so by exposing itself as a global pointer calledg_pCGASModule
, which can be used inside any scope, inside any class, and inside any file to retrieve/create any of your game's systems.CGASStartup
: This class implements CRYENGINE'sIGameStartup
interface. It is used to create and initialize all of CRYENGINE's systems and yourGame
class. This class is instantiated automatically from outside your Game DLL by the Launcher, and is expected to create and initialize all of CRYENGINE's modules and ultimately create and run your game. For now, we loadCryGameFramework.dll
, retrieve its exported factory method, and call it to instantiate an instance of theIGameFramework
interface. We then proceed to initialize it, which, in turn, loads, creates, and initializes all of CRYENGINE's modules.CPlayer
: This class implements CRYENGINE'sIActor
interface. Every CRYENGINE game needs to have a player implementation. This is the place to implement the logic that concerns the player, such as moving around the world and interacting with objects.CSmoothCamera
: This class implements CRYENGINE'sIGameObjectExtension
andIGameObjectView
interfaces. It's used to provide a view for our player so that we may see the world. TheCSmoothCamera
class implementsIGameObjectView
so that it may control a CRYENGINEIView
instance. It implementsIGameObjectExtension
so that they may be added to a game object that's in the game's world. This class is slightly advanced and it would be better to read the in-code documentation to get a clearer understanding of how this class works.IWindowEventSystem
: This interface is a completely custom interface in that it doesn't derive from any CRYENGINE interface. I created this interface to help you; by definition, it is a helper interface. Its sole purpose is to provide a mechanism for which to dispatch and handle various window events, such as window activation, closing, and various mouse/keyboard events.CScriptBind_Game
: This class implements CRYENGINE'sCScriptableBase
class. It's used to expose your game's functionality to Lua. Although not required, any game-specific functionality you want to be exposed to Lua should be added here. For now, only the ability to set the game's current spawnpoint has been exposed.
By now, you should have a good understanding of what each of the classes do and what they are used for. For a more thorough understanding, you may want to take a look at the in-code documentation.