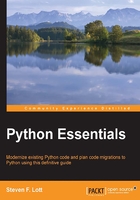
Using the built-in conversion functions
We have a number of conversion functions in the various types of data we've seen in this chapter. Each of the built-in numeric types has a proper constructor function. As with many Python functions, each of these has a number of different kinds of arguments it can handle:
int()
: Creates anint
from a wide variety of other objectsint(3.718)
for another numberint('48879')
for a string in base 10int('beef', 16)
for a string in the given base—16 in this example- The
int()
function can ignore the extra prefix characters on numbers written in Python literal syntax:int('0b1010',2)
,int('0xbeef',16)
, andint('0o123',8)
float()
: Creates afloat
from other objectsfloat(7331)
for another numberfloat('4.8879e5')
for a decimal string
complex()
: Createscomplex
values from a variety of objectscomplex(23)
creates(23+0j)
complex(23, 3)
creates(23+3j)
complex('23+2j')
creates(23+2j)
We can convert single numbers, pairs of numbers, and even some strings into Fraction
objects:
Fraction(2,3)
: This is the most common way to createFraction
objects.Fraction(2.718)
: This creates a valueFraction(765048986699563, 281474976710656)
. This shows how floating-point values are actually approximations. If we wanted a more accurate value, we should do a meaningful conversion ourselves, usingFraction(2718,1000)
, which would avoid the error bits present in many floating-point values.Fraction("3/4")
: This also works very nicely to create a properFraction
object.
When we convert a float
value to a Fraction
, the results look unusual. However, considering that float values are an approximation, the Fraction
value reveals the nature of the approximation.
We can also convert integers, strings, and floats to Decimal
objects:
Decimal(2)
: Interestingly, this producesDecimal('2')
as the result. This shows us that the preferred format forDecimal
values is strings.Decimal('2.718')
: This will produce the expected value. This is generally how we createDecimal
objects.Decimal(2.718)
: This will produce a value that reflects floating-point approximations:Decimal('2.717999999999999971578290569595992565155029296875')
. Because of this, we generally avoid creatingDecimal
objects fromfloat
objects.
We have a number of additional conversions from numbers to various kinds of strings: bin()
, oct()
, hex()
, and str()
produce strings in base 2, 8, 16, and 10 respectively. We can also use various formatting features of numbers using "{0:b}".format(x)
for binary, "{0:o}".format(x)
for octal, and "{0:x}".format(x)
for hexadecimal. If we include the "#
" modifier in the format string, we have considerable flexibility in the strings produced. For example:
>>> "{0:x}".format(12) 'c' >>> "{0:#x}".format(12) '0xc'
These functions show many different ways to create numbers from strings and create formatted strings from numbers.